|
Method
public RB.AssetStatus Setup(char unicodeStart, char unicodeEnd, Vector2i srcPos, SpriteSheetAsset spriteSheet, Vector2i glyphSize, int glyphsPerRow, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(List< char > characterList, Vector2i srcPos, SpriteSheetAsset spriteSheet, Vector2i glyphSize, int glyphsPerRow, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(char unicodeStart, char unicodeEnd, List< PackedSprite > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(char unicodeStart, char unicodeEnd, List< PackedSpriteID > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(char unicodeStart, char unicodeEnd, List< FastString > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(char unicodeStart, char unicodeEnd, List< string > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(List< char > characterList, List< PackedSprite > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(List< char > characterList, List< PackedSpriteID > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(List< char > characterList, List< FastString > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
public RB.AssetStatus Setup(List< char > characterList, List< string > glyphSprites, SpriteSheetAsset spriteSheet, int characterSpacing, int lineSpacing, bool monospaced)
|
Parameters
unicodeStart
|
char
|
First unicode character in the font
|
unicodeEnd
|
char
|
Last unicode character in the font
|
srcPos
|
Vector2i
|
Top left corner of the set of glyphs in the sprite sheet
|
spriteSheet
|
SpriteSheetAsset
|
The sprite sheet containing the font
|
glyphSize
|
Vector2i
|
Dimensions of a single glyph
|
glyphsPerRow
|
int
|
Amount of glyphs per row in the sprite sheet
|
characterSpacing
|
int
|
Spacing between characters
|
lineSpacing
|
int
|
Line spacing between lines of text
|
monospaced
|
bool
|
True if font is monospaced
|
characterList
|
List< char >
|
List of all characters in this font. If there are duplicate characters the last copy is used.
|
glyphSprites
|
List< PackedSprite >
|
List of packed sprites to use for the glyphs
|
glyphSprites
|
List< PackedSpriteID >
|
List of packed sprite ids to use for the glyphs
|
glyphSprites
|
List< FastString >
|
List of packed sprite names to use for the glyphs
|
glyphSprites
|
List< string >
|
List of packed sprites to use for the glyphs. Must be in same order as characterList
|
Returns
RB.AssetStatus
Setup status
DescriptionSetup a custom font that can be used with RB.Print. Fonts are setup for a contiguous range of unicode characters specified by unicodeStart and unicodeEnd parameters. Unicode gaps are not allowed, all glyphs in the given range must be provided, even if they are blank. Glyphs in a font can either come from a grid of sprites in a sprite sheet with the given glyphsPerRow. Glyphs from a sprite pack can be explicitly listed with the glyphSprites parameter. The glyphSize specifies the maximum space a single glyph will take, and corresponds to the size of each grid cell when specifying glyphs by a grid of sprites. RetroBlit will automatically trim empty horizontal white space to the left and right of each glyph, unless the monospaced parameter is specified, in which case empty space is not trimmed at all, and all glyphs will occupy the same horizontal and vertical space. Printed glyphs are spaced out with 1 pixel horizontal and vertical spacing by default. The spacing can optionally be altered with the characterSpacing and lineSpacing parameters. 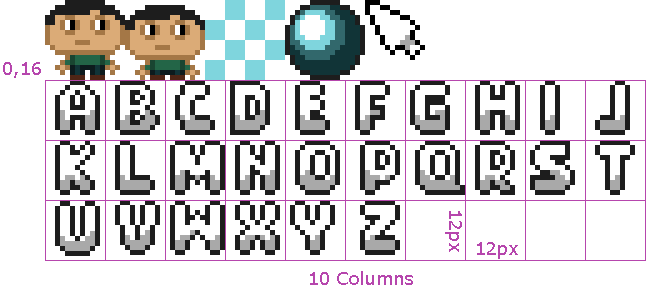 Example sprite sheet containing a grid of font glyphs. See example code below on how this font could be loaded. ExampleSpriteSheetAsset spritesheetMain = new SpriteSheetAsset(); FontAsset retroFont = new FontAsset();
void Initialize() { spritesheetMain.Load("spritesheets/main");
retroFont.Setup( 'A', 'Z', new Vector2i(0, 16), spritesheetMain, new Vector2i(12, 12), 10, 1, 2, false ); }
void Render() { RB.Print(retroFont, new Vector2i(0, 0), "HELLO THERE", Color.white, text);
RB.Print(retroFont, new Vector2i(0, 32), "Hello There", Color.white, text); } |
See AlsoRB.Print RB.PrintMeasure SpriteSheetAssetSee DocsFeatures - Fonts |